Extensions can communicate various information by placing info blocks
(also referred to as widgets) to the administrator’s and customers’
Home pages in Plesk.
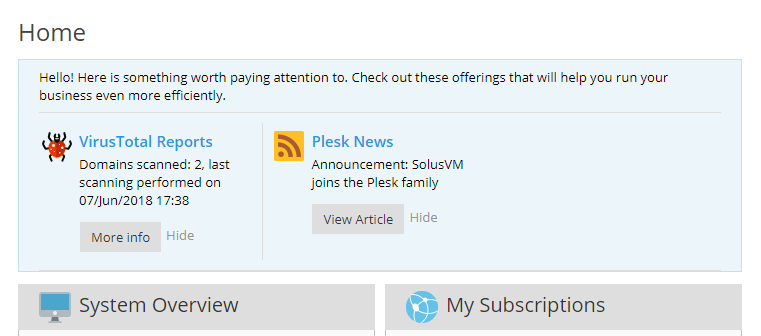
To do that, include in your extension a class that extends
pm_Promo_AdminHome
or
pm_Promo_CustomerHome
and overrides the necessary methods. All classes that extend
pm_Promo_AdminHome
and pm_Promo_CustomerHome
are loaded when a
user navigates to the Home page.
Note: Make sure to follow the Class Naming Conventions for
the classes you implement. Otherwise, the classes may not be properly
loaded.
For example, in an extension with an ID "my-module"
, a new class
implementing an info block for the administrator’s Home page should
be named Modules_MyModule_Promo_AdminHome
. Its code should be placed
in the file plib/library/Promo/AdminHome.php
.
“Plesk News” info block on the screenshot above is displayed by the
sample extension “Panel News”. Its source code is available here:
https://github.com/plesk/ext-panel-news
Example: defining an info block
This example demonstrates an implementation of a info block. It uses the
following methods:
Use the following code:
<?php
class Modules_MyModule_Promo_AdminHome extends pm_Promo_AdminHome
{
public function getTitle()
{
return 'Add Block Title Here';
}
public function getText()
{
return 'Add your text here...';
}
public function getButtonText()
{
return 'View';
}
public function getButtonUrl()
{
return 'http://example.com';
}
public function getIconUrl()
{
pm_Context::init('my-module');
return pm_Context::getBaseUrl() . '/images/icon.png';
}
}
Note: When you need to use the functions provided by the Extensions API,
you need to define context in the method body by calling
pm_Context::init('module ID')
.
The getButtonText()
and getIconUrl()
methods are optional. If
you omit these methods, Plesk will use the default button caption
(“Learn more”) and icon.